
Given a dictionary D, the syntax D returns the value of key x (if it exists) or throws an error, and D = y stores the key-value pair x => y in D (replacing any existing value for the key x). For example, Dict(i => f(i) for i = 1:10). this example creates a Dict("A"=>1, "B"=>2).ĭictionaries may also be created with generators. This call will attempt to infer type information from the keys and values (i.e. Like Dict it uses hash for hashing and isequal for equality, unlike Dict it does not convert keys on insertion.ĭicts can be created by passing pair objects constructed with => to a Dict constructor: Dict("A"=>1, "B"=>2). WeakKeyDict is a hash table implementation where the keys are weak references to objects, and thus may be garbage collected even when referenced in a hash table. IdDict is a special hash table where the keys are always object identities. Define these two functions for custom types to override how they are stored in a hash table. Its implementation uses hash as the hashing function for the key, and isequal to determine equality. See also: axes, firstindex, eachindex, prevind.ĭict is the standard dictionary. The syntaxes A and A lower to A and A, respectively. If d is given, return the last index of collection along dimension d. Note that the elements are not reordered if you use an ordered collection. Future versions of Julia might change the algorithm. Parallelism will be easier if the reduction can be executed in groups.
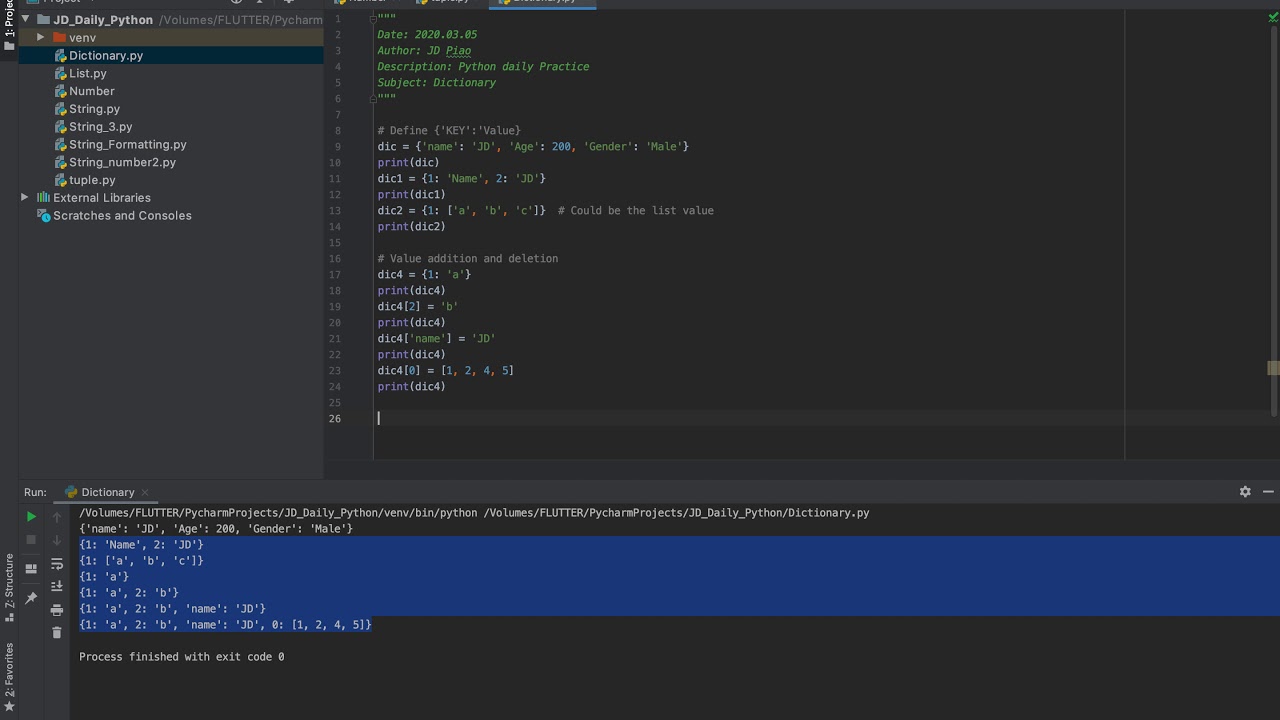
Use foldl or foldr instead for guaranteed left or right associativity. This means that you can't use non-associative operations like - because it is undefined whether reduce(-,) should be evaluated as (1-2)-3 or 1-(2-3). The associativity of the reduction is implementation dependent. There are efficient methods for concatenating certain arrays of arrays by calling reduce( vcat, arr) or reduce( hcat, arr). Reductions for certain commonly-used operators may have special implementations, and should be used instead: maximum (itr), minimum (itr), sum (itr), prod (itr), any (itr), all (itr). when op is one of +, *, max, min, &, |) when Julia can determine the neutral element of op. It is unspecified whether init is used for non-empty collections.įor empty collections, providing init will be necessary, except for some special cases (e.g. If provided, the initial value init must be a neutral element for op that will be returned for empty collections. Reduce the given collection itr with the given binary operator op. See also: insorted, contains, occursin, issubset. To get a vector indicating whether each value in items is in collection, wrap collection in a tuple or a Ref like this: in.(items, Ref(collection)) or items. For example, if both arguments are vectors (and the dimensions match), the result is a vector indicating whether each value in collection items is in the value at the corresponding position in collection. ∈ collection, both item and collection are broadcasted over, which is often not what is intended. When broadcasting with in.(items, collection) or items. For the collections mentioned above, the result is always a Bool. To test for the presence of a key in a dictionary, use haskey or k in keys(dict). For example, Sets check whether the item isequal to one of the elements Dicts look for key=>value pairs, and the key is compared using isequal. Some collections follow a slightly different definition.
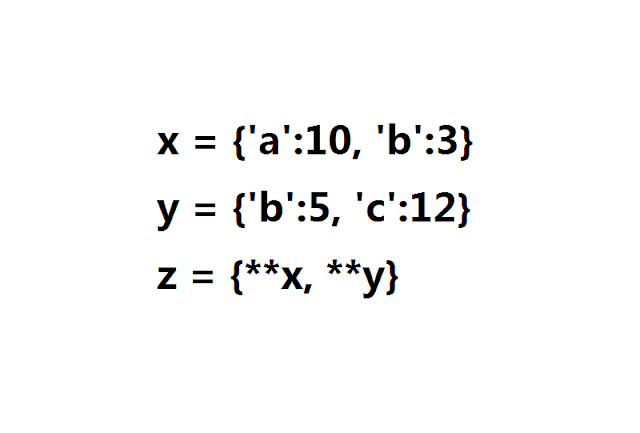
Returns a Bool value, except if item is missing or collection contains missing but not item, in which case missing is returned ( three-valued logic, matching the behavior of any and =).

Instrumenting Julia with DTrace, and bpftraceĭetermine whether an item is in the given collection, in the sense that it is = to one of the values generated by iterating over the collection.Reporting and analyzing crashes (segfaults).
#Python merge dictionaries same keys code#
